TitledBorder
ここではTitledBorderの使い方について見ていきます。TitledBorderは複合型のボーダーです。基本となるボーダーと組み合わせて、指定した位置にタイトルのような文字列を描くことができるBorderクラスです。
まずTitledBorderのクラス図を見て下さい。
java.lang.Object javax.swing.border.AbstractBorder javax.swing.border.TitledBorder public class TitledBorder extends AbstractBorder
見た目は下記のようになります。
コンストラクタ
TitledBorderではコンストラクタは6つ用意されています。
コンストラクタ |
---|
TitledBorder(Border border) 指定されたボーダと空のタイトルで、TitledBorder のインスタンスを生成します。 |
TitledBorder(Border border, String title) 指定されたボーダとタイトルで TitledBorder のインスタンスを生成します。 |
TitledBorder(Border border, String title, int titleJustification, int titlePosition) 指定されたボーダ、タイトル、タイトルの位置揃え、およびタイトルの配置で、TitledBorder のインスタンスを生成します。 |
TitledBorder(Border border, String title, int titleJustification, int titlePosition, Font titleFont) 指定されたボーダ、タイトル、タイトルの位置揃え、タイトルの配置、およびタイトルのフォントで、TitledBorder のインスタンスを生成します。 |
TitledBorder(Border border, String title, int titleJustification, int titlePosition, Font titleFont, Color titleColor) 指定されたボーダ、タイトル、タイトルの位置揃え、タイトルの配置、タイトルのフォント、およびタイトルのカラーで、TitledBorder のインスタンスを生成します。 |
TitledBorder(String title) TitledBorder インスタンスを作成します。 |
コンストラクタは6つありますが、基本となるボーダーと、タイトルの位置やフォント及びカラーなどを指定します。
5番目のコンストラクタを見てみましょう。
public TitledBorder(Border border, String title, int titleJustification, int titlePosition, Font titleFont, Color titleColor)
指定されたボーダ、タイトル、タイトルの位置揃え、タイトルの配置、タイト ルのフォント、およびタイトルのカラーで、TitledBorder のインスタンスを 生成します。 パラメータ: border - ボーダ title - ボーダに表示するタイトル titleJustification - タイトルの位置揃え titlePosition - タイトルの位置 titleFont - タイトルのフォント titleColor - タイトルのカラー
まずは基本となるBorderクラスを指定します。ここには今まで出てきたようなEtchedBorderやLineBorderなどを指定します。
次にタイトルの位置揃えです。タイトルの文字を左寄せ、中央、右寄せのどの位置に表示するかを指定します。指定可能な値は下記のどれかです。
TitledBorder.LEFT 左寄せ TitledBorder.CENTER 中央 TitledBorder.RIGHT 右寄せ TitledBorder.LEADING TitledBorder.TRAILING
次はタイトルの位置です。基本となるボーダーの線のどの位置にタイトルを表示するかを指定します。例えば線上にタイトルを表示したり、線の上に乗っかるようにタイトルを表示したり指定できます。指定可能な値は下記の通りです。
TitledBorder.ABOVE_TOP トップラインより上 TitledBorder.TOP トップラインの中央 TitledBorder.BELOW_TOP トップラインより下 TitledBorder.ABOVE_BOTTOM ボトムラインより上 TitledBorder.BOTTOM ボトムラインの中央 TitledBorder.BELOW_BOTTOM ボトムラインより下
また、タイトルのフォントやカラーも指定可能です。
実際の記述方法は下記のようになります。
LineBorder inborder = new LineBorder(Color.red, 2); TitledBorder border = new TitledBorder(inborder, "Sports", TitledBorder.LEFT, TitledBorder.TOP); JButton btn1 = new JButton("Tennis"); btn1.setPreferredSize(new Dimension(100,100)); btn1.setBorder(border);
メソッドでの設定
何故かTitledBorderクラスだけはメソッドが用意されています。その為、コンストラクタで設定する値は、メソッドでも指定が可能です。各メソッドはコンストラクタでの設定と同じですので、説明は省略します。
setBorderメソッド
public void setBorder(Border border)
タイトル付きボーダのボーダを設定します。 パラメータ: border - ボーダ
setTitleメソッド
public void setTitle(String title)
タイトル付きボーダのタイトルを設定します。 パラメータ: title - タイトル
setTitlePositionメソッド
public void setTitlePosition(int titlePosition)
タイトル付きボーダのタイトル位置を設定します。 パラメータ: titlePosition - ボーダの位置
setTitleJustificationメソッド
public void setTitleJustification(int titleJustification)
タイトル付きボーダのタイトル位置揃えを設定します。 パラメータ: titleJustification - ボーダの位置揃え
setTitleFontメソッド
public void setTitleFont(Font titleFont)
タイトル付きボーダのタイトルフォントを設定します。 パラメータ: titleFont - ボーダタイトルのフォント
setTitleColorメソッド
public void setTitleColor(Color titleColor)
タイトル付きボーダのタイトルカラーを設定します。 パラメータ: titleColor - ボーダタイトルのカラー
サンプル
では実際に試してみましょう。
import javax.swing.*; import java.awt.event.*; import java.awt.Color; import javax.swing.border.*; import java.awt.Dimension; import java.awt.Insets; public class SwingTest extends JFrame{ public static void main(String[] args){ SwingTest test = new SwingTest("SwingTest"); test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); test.setVisible(true); } SwingTest(String title){ setTitle(title); setBounds( 10, 10, 300, 200); JPanel p = new JPanel(); LineBorder inborder1 = new LineBorder(Color.red, 2); TitledBorder border1 = new TitledBorder(inborder1, "Sports", TitledBorder.LEFT, TitledBorder.TOP); JButton btn1 = new JButton("Tennis"); btn1.setPreferredSize(new Dimension(100,100)); btn1.setBorder(border1); BevelBorder inborder2 = new BevelBorder(BevelBorder.LOWERED); TitledBorder border2 = new TitledBorder(inborder2, "Sports", TitledBorder.CENTER, TitledBorder.BELOW_BOTTOM); JButton btn2 = new JButton("Golf"); btn2.setPreferredSize(new Dimension(100,100)); btn2.setBorder(border2); p.add(btn1); p.add(btn2); getContentPane().add(p); } }
実行結果は下記のようになります。
( Written by Tatsuo Ikura )
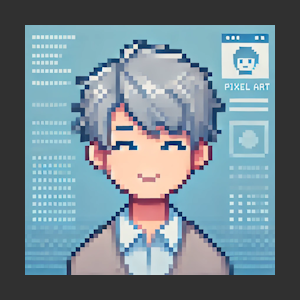
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。