コンポーネントの水平方向の表示位置を設定する
BoxLayoutで縦方向にコンポーネントを配置している場合、各コンポーネントは対象のコンテナの中で左に表示されます。(ただしデフォルトでどこに表示されるのかはコンポーネントの種類によります)。
ここでは水平方向の表示位置について設定する方法を確認します。設定するには垂直方向の表示位置を設定する場合と同じく対象のコンテナに配置される各コンポーネントに個別に設定を行います。JComponentクラスで用意されている「setAlignmentX」メソッドを使います。
public void setAlignmentX(float alignmentX)
垂直の配置方法を設定します。 パラメータ: alignmentX - 新しい垂直の配置方法
引数には水平方向の位置を表す値をfloat型の値で指定します。値は0.0fから1.0fまでの数値で指定します。0.0fであれば左端に接する位置に配置され、1.0fであれば右端に接する形で配置されます。0.5fならば中央です。
またよく使われる値がComponentクラスで定義されています。
定義された値 | 実際の値 |
---|---|
Component.LEFT_ALIGNMENT | 0.0f |
Component.CENTER_ALIGNMENT | 0.5f |
Component.RIGHT_ALIGNMENT | 1.0f |
実際の使い方は次のようになります。
JPanel p = new JPanel(); p.setLayout(new BoxLayout(p, BoxLayout.PAGE_AXIS)); JButton button1 = new JButton("button1"); button1.setAlignmentX(0.5f); JButton button2 = new JButton("button1"); button2.setAlignmentX(0.5f); p.add(button1); p.add(button2);
サンプルプログラム
では簡単なサンプルを作成して試してみます。
import javax.swing.*; import java.awt.Font; import java.awt.BorderLayout; import javax.swing.event.*; import java.util.Hashtable; public class BoxLayoutTest4 extends JFrame implements ChangeListener{ JSlider slider; JButton button1; JButton button2; JButton button3; public static void main(String[] args){ BoxLayoutTest4 frame = new BoxLayoutTest4(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setBounds(10, 10, 300, 200); frame.setTitle("タイトル"); frame.setVisible(true); } BoxLayoutTest4(){ button1 = new JButton("Google"); button1.setAlignmentX(0.5f); button2 = new JButton("Yahoo!"); button2.setFont(new Font("Arial", Font.PLAIN, 30)); button2.setAlignmentX(0.5f); button3 = new JButton("MSN"); button3.setAlignmentX(0.5f); JPanel p = new JPanel(); p.setLayout(new BoxLayout(p, BoxLayout.PAGE_AXIS)); p.add(button1); p.add(button2); p.add(button3); Hashtable<Integer, JComponent> table = new Hashtable<Integer, JComponent>(); table.put(new Integer(0), new JLabel("0.0f ")); table.put(new Integer(5), new JLabel("0.5f ")); table.put(new Integer(10), new JLabel("1.0f ")); slider = new JSlider(0, 10); slider.setMajorTickSpacing(1); slider.setPaintTicks(true); slider.setLabelTable(table); slider.setPaintLabels(true); slider.addChangeListener(this); getContentPane().add(p, BorderLayout.CENTER); getContentPane().add(slider, BorderLayout.PAGE_END); } public void stateChanged(ChangeEvent e) { float pos = (float)(slider.getValue()) / 10.0f; button1.setAlignmentX(pos); button2.setAlignmentX(pos); button3.setAlignmentX(pos); button1.revalidate(); } }
上記をコンパイルした後で実行すると次のように表示されます。
下側にあるスライダーを動かして水平方向の位置の数値を変化させてみます。0.0fの位置まで移動させた場合は次のようになります。
また1.0fの位置まで移動させた場合は次のようになります。
( Written by Tatsuo Ikura )
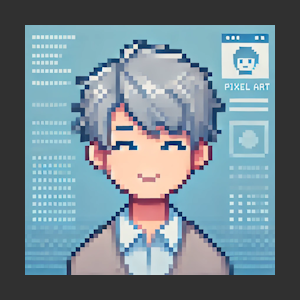
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。