選択された項目の値の取得
次に選択されている項目のIndexや実際の値を取得してみます。まずは項目が選択されているかどうかの判定と、選択されている場合の最小のIndexと最大のIndexを調べるメソッドを見てみます。
項目が選択されているかどうか調べるにはJListクラスで用意されている"isSelectionEmpty"メソッドを使います。
public boolean isSelectionEmpty()
何も選択されていない場合は true を返します。selectionModel に委譲する 簡易メソッドです。 戻り値: 何も選択されていない場合は true
選択されている項目の最小のインデックス番号と最大のインデックス番号を調べるには、JListクラスで用意されている"getMinSelectionIndex"メソッドと"getMaxSelectionIndex"メソッドを使います。
getMinSelectionIndexメソッド
public int getMinSelectionIndex()
選択されているセルの最小インデックスを返します。selectionModel に委譲 する簡易メソッドです。 戻り値: 選択されているセルの最小インデックス
getMaxSelectionIndexメソッド
public int getMaxSelectionIndex()
選択されているセルの最大インデックスを返します。selectionModel に委譲 する簡易メソッドです。 戻り値: 選択されているセルの最大インデックス
簡単なサンプルプログラムを試してみます。
import javax.swing.*; import java.awt.*; import java.awt.event.*; import javax.swing.event.*; public class JListSample extends JFrame implements ActionListener{ protected JList list; protected JLabel label; public static void main(String[] args){ JListSample test = new JListSample("JListSample"); /* 終了処理を変更 */ test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); test.setBounds( 10, 10, 250, 180); test.setVisible(true); } JListSample(String title){ setTitle(title); /* JListの初期データ */ String[] initData = {"Blue", "Green", "Red", "Whit", "Black"}; list = new JList(initData); JScrollPane sp = new JScrollPane(); sp.getViewport().setView(list); sp.setPreferredSize(new Dimension(200, 80)); JPanel p = new JPanel(); p.add(sp); getContentPane().add(p, BorderLayout.CENTER); label = new JLabel(); JButton checkButton = new JButton("Check"); checkButton.addActionListener(this); checkButton.setActionCommand("checkButton"); JPanel p2 = new JPanel(); p2.add(label); p2.add(checkButton); getContentPane().add(p2, BorderLayout.SOUTH); } public void actionPerformed(ActionEvent e){ String actionCommand = e.getActionCommand(); StringBuffer sb = new StringBuffer(); if (actionCommand.equals("checkButton")){ if (list.isSelectionEmpty()){ sb.append("Empty"); }else{ sb.append("Min:"); sb.append(list.getMinSelectionIndex()); sb.append(",Max:"); sb.append(list.getMaxSelectionIndex()); } }else{ return; } label.setText(new String(sb)); } }
実行結果は下記のようになります。
0番目と2番目を選択している場合は下記のようになります。
選択された項目のインデックス番号と内容を取得する
次に最初に選択された項目のインデックス番号と項目の内容を取得するメソッドについてです。
選択された項目のインデックス番号を取得するには"getSelectedIndex"メソッドを使います。
public int getSelectedIndex()
最初に選択されたインデックスを返すか、選択項目がない場合は -1 を返します。 戻り値: getMinSelectionIndex の値
選択された項目の値を取得するには"getSelectedValue"メソッドを使います。
public Object getSelectedValue()
最初に選択されたインデックスを返すか、選択が空の場合は null を返します。 戻り値: 最初に選択された値
では実際に試してみます。
import javax.swing.*; import java.awt.*; import java.awt.event.*; import javax.swing.event.*; public class JListSample extends JFrame implements ActionListener{ protected JList list; protected JLabel label; public static void main(String[] args){ JListSample test = new JListSample("JListSample"); /* 終了処理を変更 */ test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); test.setBounds( 10, 10, 250, 180); test.setVisible(true); } JListSample(String title){ setTitle(title); /* JListの初期データ */ String[] initData = {"Blue", "Green", "Red", "Whit", "Black"}; list = new JList(initData); JScrollPane sp = new JScrollPane(); sp.getViewport().setView(list); sp.setPreferredSize(new Dimension(200, 80)); JPanel p = new JPanel(); p.add(sp); getContentPane().add(p, BorderLayout.CENTER); label = new JLabel(); JButton indexButton = new JButton("Index"); indexButton.addActionListener(this); indexButton.setActionCommand("indexButton"); JButton valueButton = new JButton("Value"); valueButton.addActionListener(this); valueButton.setActionCommand("valueButton"); JPanel p2 = new JPanel(); p2.add(label); p2.add(indexButton); p2.add(valueButton); getContentPane().add(p2, BorderLayout.SOUTH); } public void actionPerformed(ActionEvent e){ String actionCommand = e.getActionCommand(); StringBuffer sb = new StringBuffer(); if (actionCommand.equals("indexButton")){ int index = list.getSelectedIndex(); if (index == -1){ sb.append("Empty"); }else{ sb.append("FirstIndex:"); sb.append(index); } }else if (actionCommand.equals("valueButton")){ String val = (String)list.getSelectedValue(); if (val == null){ sb.append("Empty"); }else{ sb.append("FirstValue:"); sb.append(val); } }else{ return; } label.setText(new String(sb)); } }
実行結果は下記のようになります。
2番目を選択してから0番目を選択した場合は下記のようになります。
選択した順を覚えていて、最初に選択した項目の値を返すのかと思っていたのですが、最小のIndex番号の項目を返すだけのようでした。
選択項目全部の値を取得する場合
では最後に選択されている項目全部の値を取得したい場合です。
選択されている項目のインデックス番号を全て取得するには、JListクラスで用意されている"getSelectedIndices"メソッドを使います。
public int[] getSelectedIndices()
選択されているすべてのインデックスの昇順配列を返します。 戻り値: 選択されているすべてのインデックス (昇順)
同じように選択されている項目の値を全て取得するには、JListクラスで用意されている"getSelectedValues"メソッドを使います。この場合、取得する値は選択した順番に関係無く、インデックス番号順に昇順に取得されます。
public Object[] getSelectedValues()
選択されたセルの値の配列を返します。値はインデックスの昇順に並べられます。 戻り値: 選択された値。何も選択されていない場合は空のリスト
サンプルプログラムで動作を確認します。
import javax.swing.*; import java.awt.*; import java.awt.event.*; import javax.swing.event.*; public class JListSample extends JFrame implements ActionListener{ protected JList list; protected JLabel label; public static void main(String[] args){ JListSample test = new JListSample("JListSample"); /* 終了処理を変更 */ test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); test.setBounds( 10, 10, 250, 200); test.setVisible(true); } JListSample(String title){ setTitle(title); /* JListの初期データ */ String[] initData = {"Blue", "Green", "Red", "Whit", "Black"}; list = new JList(initData); JScrollPane sp = new JScrollPane(); sp.getViewport().setView(list); sp.setPreferredSize(new Dimension(200, 80)); JPanel p = new JPanel(); p.add(sp); getContentPane().add(p, BorderLayout.CENTER); label = new JLabel("Selected List"); JButton indexButton = new JButton("Index"); indexButton.addActionListener(this); indexButton.setActionCommand("indexButton"); JButton valueButton = new JButton("Value"); valueButton.addActionListener(this); valueButton.setActionCommand("valueButton"); JPanel p2 = new JPanel(); p2.add(label); JPanel p3 = new JPanel(); p3.add(indexButton); p3.add(valueButton); getContentPane().add(p2, BorderLayout.NORTH); getContentPane().add(p3, BorderLayout.SOUTH); } public void actionPerformed(ActionEvent e){ String actionCommand = e.getActionCommand(); StringBuffer sb = new StringBuffer(); if (actionCommand.equals("indexButton")){ int[] index = list.getSelectedIndices(); if (index.length == 0){ sb.append("Empty"); }else{ sb.append("Index:"); for (int i = 0 ; i < index.length ; i++){ sb.append(index[i]); sb.append(" "); } } }else if (actionCommand.equals("valueButton")){ Object[] val = list.getSelectedValues(); if (val.length == 0){ sb.append("Empty"); }else{ sb.append("Value:"); for (int i = 0 ; i < val.length ; i++){ sb.append(val[i]); sb.append(" "); } } }else{ return; } label.setText(new String(sb)); } }
実行結果は下記のようになります。
選択した状態でIndexボタンを押した場合は下記のようになります。
選択した状態でValueボタンを押した場合は下記のようになります。
( Written by Tatsuo Ikura )
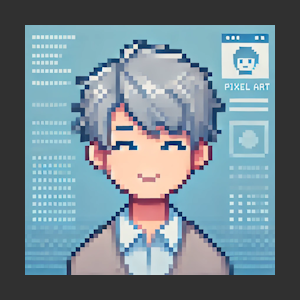
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。